Hi Ryder!
So to preface my following comments… I see Hype as a construction kit and often there are several ways to accomplish the same goal. The approach You pick is usually based on the task at hand, familiarity with the Hype interface, and possibly skill with JavaScript. One does not need to be a JavaScript guru to utilize this valuable tool. A basic understanding of JavaScript will prove useful for a myriad of tasks that the Hype interface can not accomplish on its own or without great difficulty.
Also, I find specific systemic nomenclature to be important unless the project is dead simple involving just a few objects; in dealing with 50 objects as postulated in your post above I find this sort of discipline to be a must.
I’ve changed the Hype Demo file to a more simplified version (v4) for this explanation compared to v3 in my post above: tooltip_JHSv4.hype.zip (34.7 KB)
In this Demo:
It does not matter what the Scene name is called.
It does matter what the graphic object & its associated tooltip element classes are named with the restricting aspect being that the last two characters of these names are a match for each other e.g. “frontDoor_01” and “tooltip_01”; or “redBlanket-01” and “redblanket_tTip-01”. It is the last characters “01” & “02 etc. that will be the connecting information between the two elements. It could be letters instead of numbers if You wanted. Digits are more utilitarian for me. (Class names are assigned in Hype’s “Identity” panel.)
When a “class name” is assigned to an element it also gets an index number (i.e. [0] , [1] ) associated with it according to the layout order in the Hype “Layout” panel. The index numbers always start with “0” not “1”. You can not see these class index numbers in the Hype interface. A simplified class read out for the “Vector Shape” in the “iPhone” layout would look like “shape01[0]”. In the “Desktop” layout it would look roughly like “shape01[1]”, with the last digit in brackets being the index number for the element's class name.
======================================
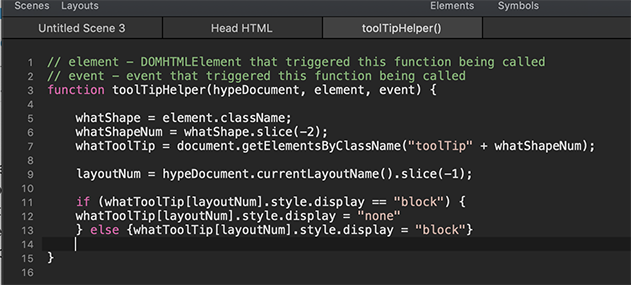
======================================
So if we look at our code we see that first we get the class name of the “element” that fires the function “toolTipHelper” via the “Mouse Over” event. “whatShape” is a “variable” that is assigned this information.
whatShape = element.className; // e.g. shape01
Next we trim off the last two digits of this class name and use it to hook into the matching tool tip. For this operation we use the slice method.
whatShapeNum = whatShape.slice(-2); // e.g. 01
whatToolTip = document.getElementsByClassName("toolTip" + whatShapeNum); // e.g. toolTip01
And finally we use the last digit of the Layout schema to quickly establish the index number for the particular class name we have identified to this point (toolTip01). We employ the same method that we did above to isolate the last digit of the current layout name - the slice method.
layoutNum = hypeDocument.currentLayoutName().slice(-1); // e.g. “Desktop_1” layout, last character = "1"
Now we have all the information we need to show or hide the matching tool tip: Class name “toolTip01” with an index number of [1] (in the Desktop layout). To accomplish this task we will employ the “if/else” conditional.
If the tooltip is visible (display == block) then hide it {...display == “none”}… else if it is hidden show it {...display == block”}
if (whatToolTip[layoutNum].style.display == "block") {
whatToolTip[layoutNum].style.display = "none"
} else {whatToolTip[layoutNum].style.display = "block"}
Note: You can use this code without alteration as long as You stick to the premises discussed above. Though it is far better to understand what is going on. For instance I took a short cut to help keep things simple for this explanation by using the layout name to establish the index number for a given tool tip class name. The two links in my previous post above discuss a more comprehensive way to accomplish this goal.